- Forum
- Docs
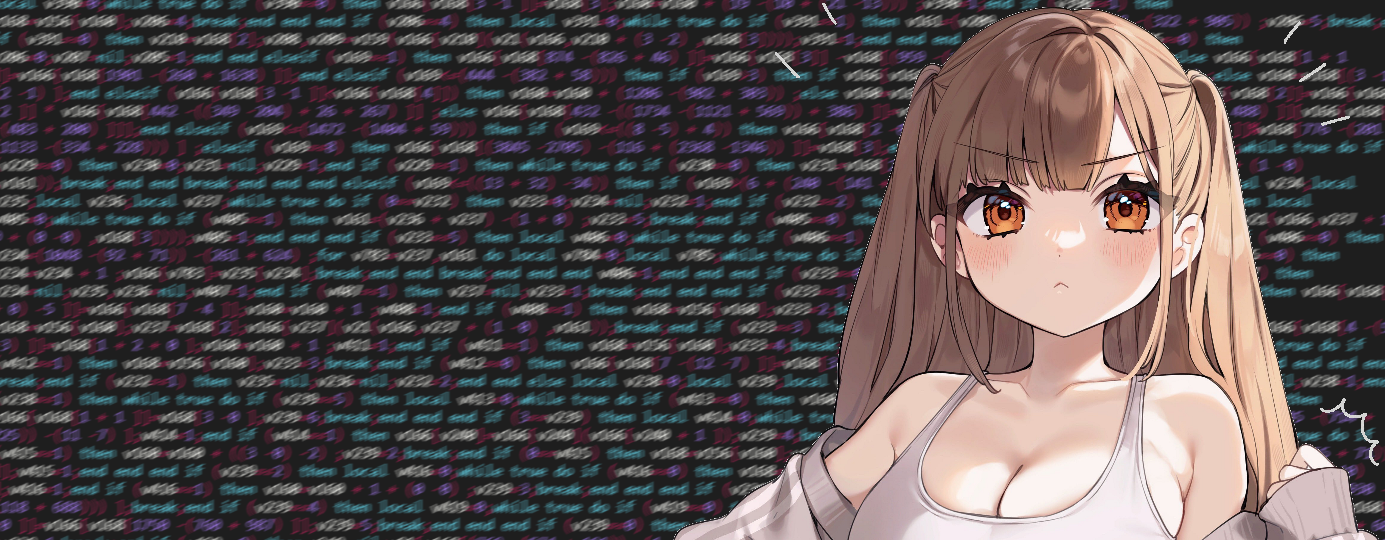
Community
📄Docs
Getting Started
The Lua obfuscator API provides a straightforward way to obfuscate Lua scripts.
To begin, you'll need to obtain an API key for authentication, that you can get here.
By sending HTTP requests to designated endpoints, you can seamlessly integrate script obfuscation into their workflows.
/obfuscator/newscript
- uploads your scripts/obfuscator/obfuscate
- obfuscates the uploaded script
Responses typically include the obfuscated Lua script or relevant information such as the sessionId, used to apply obfuscation methods on your uploaded script.
HTTP Requests
/newscript
This endpoint initiates a new obfuscation session for a Lua script, wich will return the created sessionId. Your script is placed in the requests body.
Headers:
apikey: YOUR_APIKEY
content-type: text
Body:
local test = "Hello World"
print(test)
Response:
{
"message": null
"sessionId": "..."
}
/obfuscate
This endpoint is used to send obfuscation method requests to the Lua script using the provided sessionId.
Headers:
apikey: YOUR_APIKEY
sessionId: SESSION_ID
content-type: application/json
Body:
{
"MinifiyAll":true,
"CustomPlugins": {
...
}
}
The CustomPlugins is where the Plugins go. You write their name followed by the requested arguments.
Example: CustomPlugins: { "DummyFunctionArgs": [ 6, 9 ] }
Response:
{
"message": null
"code": "..."
"sessionId": null
}
Examples
Creating a script / session
Curl:
Copy
curl -X POST https://api.luaobfuscator.com/v1/obfuscator/newscript \
-H "content-type: application/json" \
-H "apikey: test" \
-d 'print("test")'
Example Response:
Copy
{
"message": null
"sessionId": "2v64HYPfQ89K24Db084hw3iBx...tz8PF6l20vzAQxL2G0bHJ6Zqz3j"
"code": null
}
Obfuscating a script
Curl:
Copy
curl -X POST https://api.luaobfuscator.com/v1/obfuscator/obfuscate \
-H "content-type: application/json" \
-H "apikey: test" \
-H "sessionId: 2v64HYPfQ89K24Db084hw3iBx...tz8PF6l20vzAQxL2G0bHJ6Zqz3j" \
-d '{ "MinifiyAll": true, "Virtualize": true }'
Example Response:
Copy
{
"message": null
"code": "local v0=string.char;local v1=string.byte;local v2=..."
"sessionId": null
}
Plugins
- LPH_ENCSTR
- LPH_ENCNUM
- LPH_JIT, LPH_JIT_MAX
- LPH_NO_VIRTUALIZE
- LPH_NO_UPVALUES
- LPH_OBFUSCATED*
- 1 - Tinkr
- 2 - NoName
- 3 - Daemonic
- 102 - Generic / No vendo
BasicIntegrity
Description:
EXPERIMENTAL
idk I forgot what this one did
Arguments: Boolean
CachedEncryptStrings
Description:
Encrypts all* the strings in the script and caches them in a table. Caching is done at startup.
*some strings with special characters might not be included
Arguments: Int32[]
The array of Int32 represents the percentage (max 100) of random selected nodes to by affected.
CallRetAssignment
Description:
Takes a normal assignment or declaration and wraps it into an anonymous function call which returns the value instead.
Arguments: Int32[]
The array of Int32 represents the percentage (max 100) of random selected nodes to by affected.
Example:
local foo = 42
local foo = (function()
return 42;
end)();
ControlFlowFlattenV1AllBlocks
Description:
Version 1 Control Flow Obfuscation. Injects basic while loops with a state counter.
Arguments: Int32[]
The array of Int32 represents the percentage (max 100) of random selected nodes to by affected.
Example:
local foo = 42
local bar = 6
local foo
local bar
local FlatIdent_123 = 0
while true do
if FlatIdent_123 == 0 then
foo = 42
FlatIdent_123 = 1
end
if FlatIdent_123 == 1 then
bar = 6
break
end
end
ControlFlowFlattenV2AllBlocks
Description:
EXPERIMENTAL
UNFINISHED
Arguments: Int32[]
The array of Int32 represents the percentage (max 100) of random selected nodes to by affected.
ConstMaker
Description:
Used with macro LPH_CONST() to turn a variable into a constant.
For example:
local x = LPH_CONST(123);
print(x-1);
Will obfuscate into just "print(123-1);
".
Arguments: Boolean
Example:
local foo = LPH_CONST(42)
print(foo - 1)
print(42 - 1)
DisableLuraphMacros
Description:
Will remove a bunch of LPH_* macro's to provide compatibility with certain scripts.
This includes:
*will be replace with constant 'true'
Arguments: Boolean
DummyFunctionArgs
Description:
Injects random arguments into local functions and their callers.
NOTE: Beta only
Arguments: Int32[2]
The array of Int32 expects exactly 2 values which represents 'min' and 'max' respectively. By default it is set to min 1, max 3.
Example:
local function foo(a, b)
return a + b
end
local function foo(a, b, OBF_FUNC_PARAM_1, OBF_FUNC_PARAM_2)
return a + b
end
EncryptFuncDeclaration
Description:
Turns the declaration of a (global) function into an encrypted string if possible.
Example: 'function foo() end' turns into '_G[strdec(a, b)] = function() end'
Arguments: Boolean
EncryptStrings
Description:
Encrypts all* the strings
*some strings with special characters might not be included
Arguments: Int32[]
The array of Int32 represents the percentage (max 100) of random selected nodes to by affected.
Arguments: Int32[]
local foo = "Hello World!"
local obf_stringchar = string.char;
local obf_stringbyte = string.byte;
local obf_stringsub = string.sub;
local obf_bitlib = bit32 or bit;
local obf_XOR = obf_bitlib.bxor;
local obf_tableconcat = table.concat;
local obf_tableinsert = table.insert;
local function LUAOBFUSACTOR_DECRYPT_STR_0(LUAOBFUSACTOR_STR, LUAOBFUSACTOR_KEY)
local result = {};
for i = 1, #LUAOBFUSACTOR_STR do
obf_tableinsert(result, obf_stringchar(obf_XOR(obf_stringbyte(obf_stringsub(LUAOBFUSACTOR_STR, i, i + 1)), obf_stringbyte(obf_stringsub(LUAOBFUSACTOR_KEY, 1 + (i % #LUAOBFUSACTOR_KEY), 1 + (i % #LUAOBFUSACTOR_KEY) + 1))) % 256));
end
return obf_tableconcat(result);
end
local foo = LUAOBFUSACTOR_DECRYPT_STR_0("\249\198\215\41\233\251\240\17\195\207\223\100", "\126\177\163\187\69\134\219\167");
FuncChopper
Description:
Splits a local defined function into multiple functions if possible.
Arguments: Boolean
JunkifyAllIfStatements
Description:
Injects opaque conditions into the if statement.
Arguments: Int32[]
The array of Int32 represents the percentage (max 100) of random selected nodes to by affected.
JunkifyBlockToIf
Description:
Turns do/end blocks into opaque if statements.
Arguments: Int32[]
The array of Int32 represents the percentage (max 100) of random selected nodes to by affected.
MakeGlobalsLookups
Description:
Turns all the globals explicity into these kind if lookups: _G['foo']
Arguments: Boolean
MixedBooleanArithmetic
Description:
Mutates literals into mixed boolean arithmerics
Very slow as it falls back on bit/bit32 using bor, bxor, band function calls! Also the MBAs itself are basic pattern matched
Arguments: Int32[]
The array of Int32 represents the percentage (max 100) of random selected nodes to by affected.
Minifier
Description:
Rename the local variables to v0, v1, v2, etc...
If you want everything on a single line, make sure to set "MinifiyAll:true" in the root node of the config.
Arguments: Boolean
The Boolean argument indicates if the code is part of multiple files. If set to false, it assumes the code is standalone and renames lookup variables that may conflict with multiple files (e.g., foo.bar becomes v0.v0, instead of v0.bar).
NOTE: The minifier is enabled regardless if the argument is set to true/false!
NOTE2: If you don't know what any of that means, just set it to true.
Example:
local foo = 42
local v0 = 42
Minifier2
Description:
Similar to the original Minifier, but will try to re-use variable names after their lifetime is over.
Arguments: Boolean
The Boolean argument indicates if the code is part of multiple files. If set to false, it assumes the code is standalone and renames lookup variables that may conflict with multiple files (e.g., foo.bar becomes v0.v0, instead of v0.bar).
NOTE: The minifier2 is enabled regardless if the argument is set to true/false!
NOTE2: If you don't know what any of that means, just set it to true.
Example:
local foo = 42
local v0 = 42
MutateAllLiterals
Description:
Mutates all numeric literals into basic +/- binary nodes.
These are cute as they come with zero performance impact due to the Lua compiler providing constant folding at compile time :3
Arguments: Int32[]
The array of Int32 represents the percentage (max 100) of random selected nodes to by affected.
Example:
local foo = 42
local bar = 69
local foo = 39 + 3
local bar = 25 + (84 - 40)
Live Example:
locala=
420400+20
MutateAllLiteralsIntoDeclarations
Description:
I don't know what I smoked here, but this turns EVERY literal into a local variable.
This will very likely break your script, and also exceeds the 200 local variable limit in no time!
Arguments: Int32[]
The array of Int32 represents the percentage (max 100) of random selected nodes to by affected.
OptimizeDeadLocals
Description:
Replaces every declaration (e.g. local foo = bar
) that is never used.
Arguments: Boolean
VirtualizeLua51
Description:
Creates a Lua 5.1 runtime and executes a 'compiled' version of the script provided inside this unique interpreter. Effectively 'virtualizing' the Lua 5.1 script into another Lua 5.1 instance.
Arguments: Object
The argument is currently unused, but might be replaced with a configuration of the VM in the near future.
WowPacker
Description:
Wraps the code into a 'loadstring' call that performs some basic XOR decryption pre loading.
Arguments: Int32
Support for the following third-party vendors is added:
RevertAllIfStatements
Description:
This will invert all if statements to create funny looking ones
They tends to give inf loops, especially when control flow obfuscation is applied.
Arguments: Int32[]
The array of Int32 represents the percentage (max 100) of random selected nodes to by affected.
RewriteToLua51
Description:
Transpiles the current script to something that is compatible with Lua 5.1
The transpiler takes care of 'continue', compounding operators like '+=' and some other fancy things.
NOTE: it is not capable yet of dealing with goto labels!
Arguments: Boolean
SwizzleLookups
Description:
Swizzle lookups, will turn foo.bar into foo['bar']. (very nice with string encryption)
Arguments: Int32[]
The array of Int32 represents the percentage (max 100) of random selected nodes to by affected.
TableIndirection
Description:
Replaces local variables with a table, each varaible is mapped to a table index.
Arguments: Int32
The Int32 represents the percentage (max 100) of random selected nodes to by affected.
Example:
local foo = 42
local TABLE_TableIndirection = {};
TABLE_TableIndirection["foo%0"] = 42
WriteLuaBit32
Description:
Addes the 'bit32' in pura Lua for compatibility
Very slow, so only use if needed
Arguments: Boolean
Please note that everything marked in yellow is exclusive for Beta testers & Discord server boosters.
Extras
Seed
Description:
(This goes into the root config node)
Provide seed (Int32) to have a determenistic randomness. The seed, along with an identical config and Lua script, can be used to generate the exact same obfuscated output (handy for debugging).
MinifiyAll
Description:
(This goes into the root config node)
Set this variable to true to have the code minified. This results in all code on a single line, no comments. (Local variables remain untouched)
Virtualize
Description:
(This goes into the root config node)
Enable this to have the final code virtualized.
The virtualizer is optimized for Lua 5.1 and may fail on other versions, try to 'RewriteToLua51' beforehand!